TypeError: Tensors in List Passed to 'Concat' Must Have Same Data Type – TensorFlow Fix
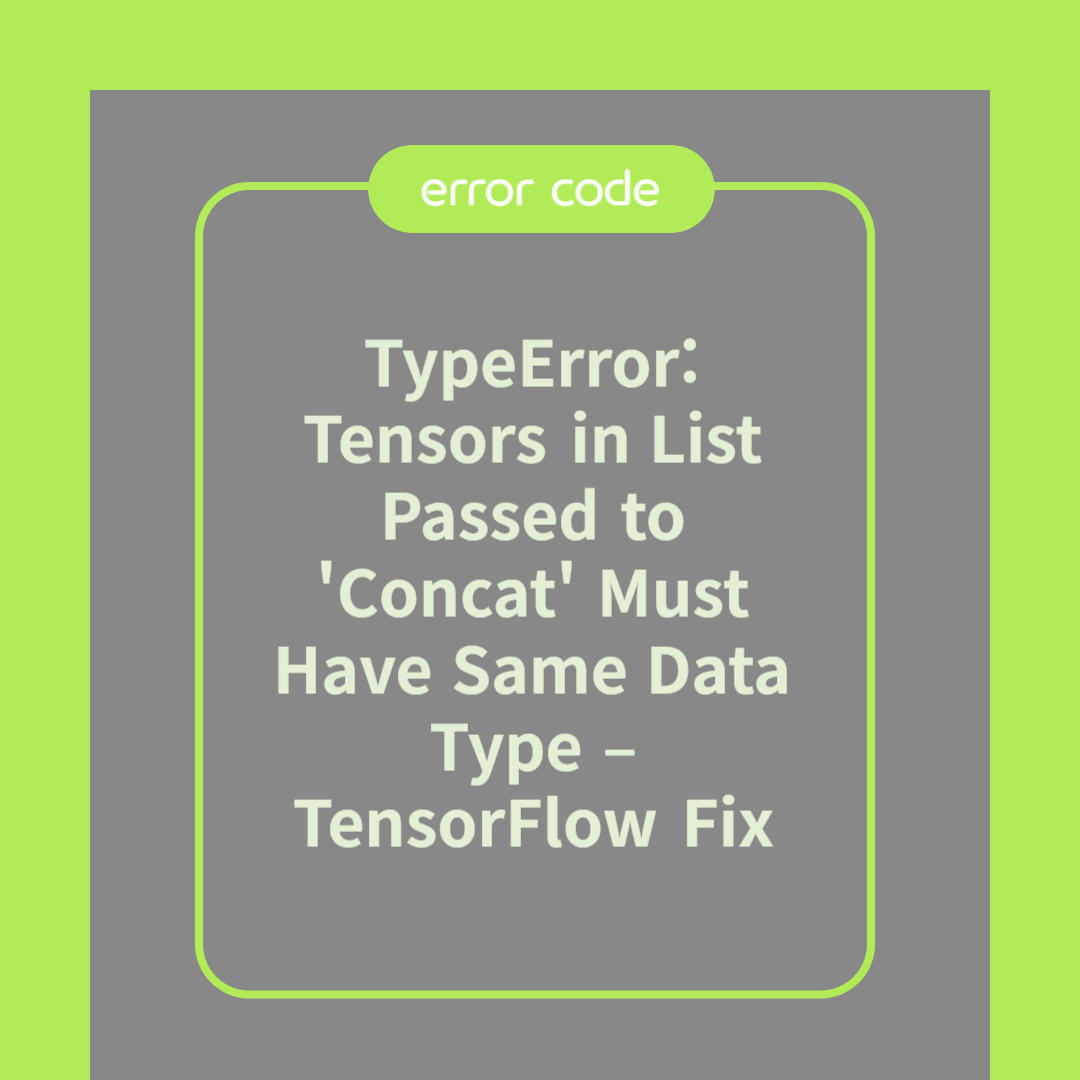
Understanding the TensorFlow Error
The error message "TypeError: Tensors in list passed to 'Concat' must have same data type" is a common issue encountered by developers using TensorFlow. This error occurs when you attempt to concatenate tensors that have different data types. In TensorFlow, operations such as tf.concat() require all tensors in the list to have the same data type, such as float32, int32, etc. For example, if you try to concatenate a tensor of type float32 with a tensor of type int32, this error will arise. Understanding this helps in debugging TensorFlow code effectively.
Common Causes of the Error
There are several reasons why this TypeError might occur. One common cause is mistakenly mixing different data types within the same tensor operation. For instance, when loading data, one might inadvertently load some data as integers and others as floats. Another possible cause is when tensors are created through operations that change their data type. For example, using tf.cast() incorrectly can lead to mismatched data types. Checking the data type of each tensor using tensor.dtype before concatenation can help prevent this error.
How to Fix the TensorFlow Error
Fixing this TensorFlow error involves ensuring that all tensors to be concatenated have the same data type. You can achieve this by explicitly casting tensors to a common data type using tf.cast(). For example, if you have a tensor a with dtype float32 and tensor b with dtype int32, convert b to float32 using b = tf.cast(b, tf.float32) before concatenating. It is also good practice to define a consistent data type for tensors when they are created, ensuring all related operations use this type.
Frequently Asked Questions (FAQ)
Q: Can I concatenate tensors of different shapes?
A: Yes, tensors with different shapes can be concatenated along a specified axis, as long as the data types match and the other dimensions are compatible.
Q: What if I don't know the data types of my tensors?
A: Use tensor.dtype to check the data type of any tensor before performing operations that require type matching.
Q: Is there a performance impact when casting tensors?
A: Yes, casting tensors can introduce a slight performance overhead, so it's best to ensure tensors have the correct type from the start.
In summary, ensuring tensors have matching data types before concatenating them in TensorFlow can prevent the "TypeError" and make your code run smoothly. Thank you for reading. Please leave a comment and like the post!