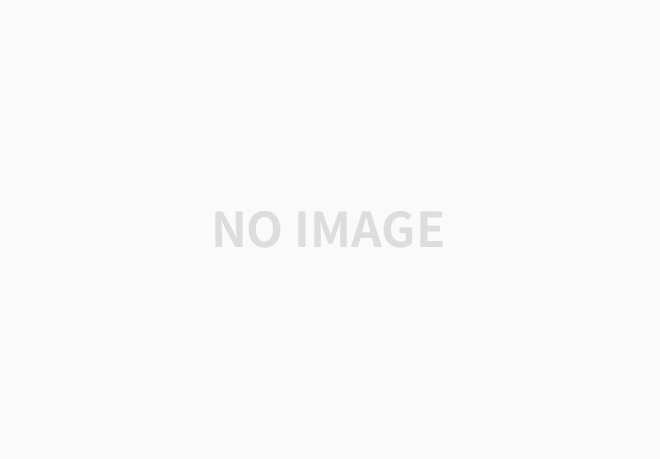
RuntimeError: Attempting to Use Uninitialized Value – TensorFlow Fix
Understanding the Error
The RuntimeError: Attempting to Use Uninitialized Value in TensorFlow can be perplexing for many developers. This error typically occurs when you attempt to use a TensorFlow variable before initializing it. In TensorFlow, variables need to be initialized explicitly using tf.global_variables_initializer()
. For example, consider a simple TensorFlow program where a variable is defined but not initialized:
import tensorflow as tf
variable = tf.Variable([1, 2, 3])
with tf.Session() as session:
print(session.run(variable))
This code will trigger the runtime error because the variable is not initialized. To fix the error, you should initialize the variables before running the session.
Steps to Resolve the Error
To resolve the RuntimeError, follow these steps. First, make sure that you initialize all your variables after their declaration and before using them in a session. You can do this using the tf.global_variables_initializer()
function. Here's an example:
import tensorflow as tf
variable = tf.Variable([1, 2, 3])
init = tf.global_variables_initializer()
with tf.Session() as session:
session.run(init)
print(session.run(variable))
In this example, the init operation is run in the session, which initializes the variable before it's used. Ensure that you include this initialization step in your TensorFlow scripts to avoid the runtime error.
Common Mistakes and How to Avoid Them
One common mistake is forgetting to run the initializer operation. Even if you have declared it, you must execute it within a session. Another mistake is misunderstanding the scope of variables. If you're using variable scopes, ensure that the initializer is called in the correct context. Consider this example:
import tensorflow as tf
with tf.variable_scope("scope"):
variable = tf.get_variable("variable", [1, 2, 3])
init = tf.global_variables_initializer()
with tf.Session() as session:
session.run(init)
print(session.run(variable))
Here, the variable is initialized within its scope, and the initializer is executed in the session. Make sure to properly manage scopes and initializations to prevent errors.
Frequently Asked Questions (FAQ)
Q1: Can I initialize variables partially?
A: Yes, you can initialize specific variables instead of all using session.run(variable.initializer)
.
Q2: What if I use Keras with TensorFlow?
A: Keras manages variable initialization automatically, but make sure to check your version compatibility with TensorFlow.
Q3: How do I check if a variable is initialized?
A: You can use tf.report_uninitialized_variables()
to identify uninitialized variables.
In summary, always initialize your TensorFlow variables to avoid runtime errors. Thank you for reading. Please leave a comment and like the post!